So You Have a Math PhD and Want to Beat the S&P 500
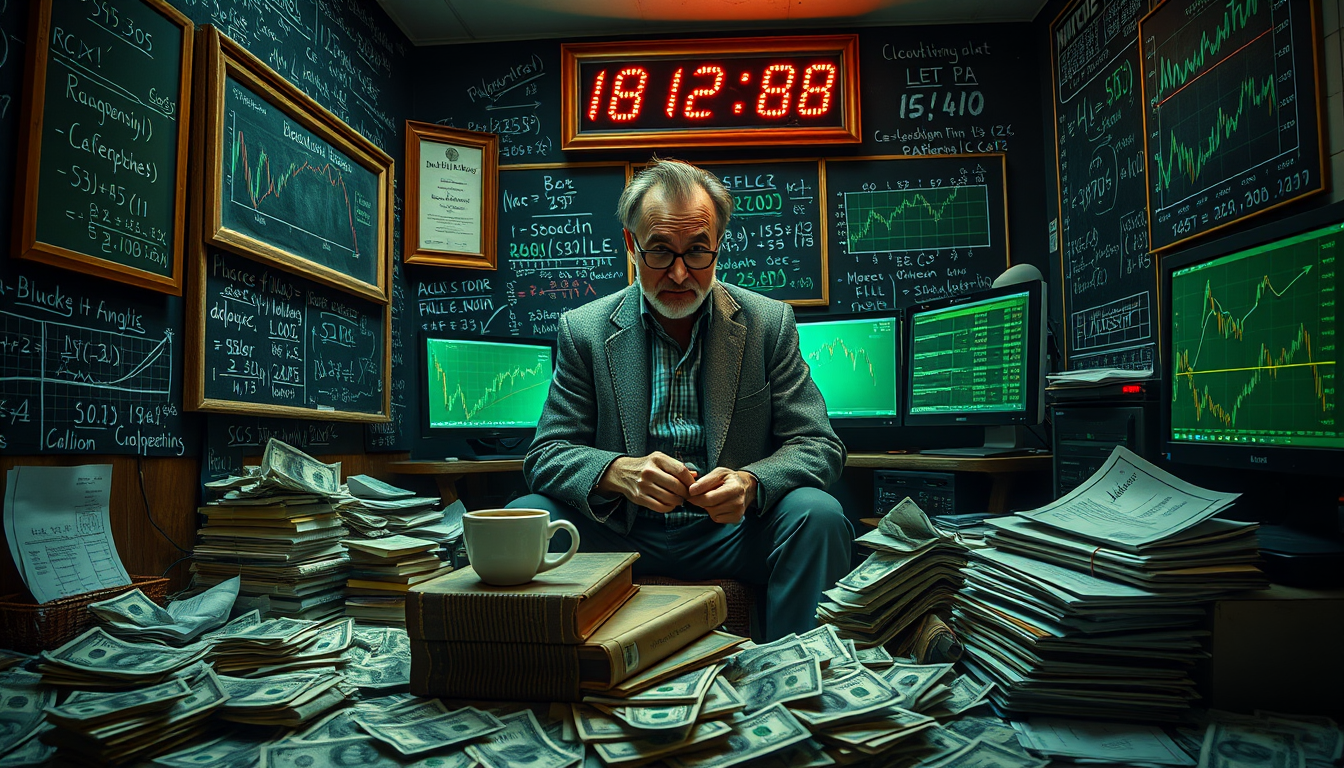
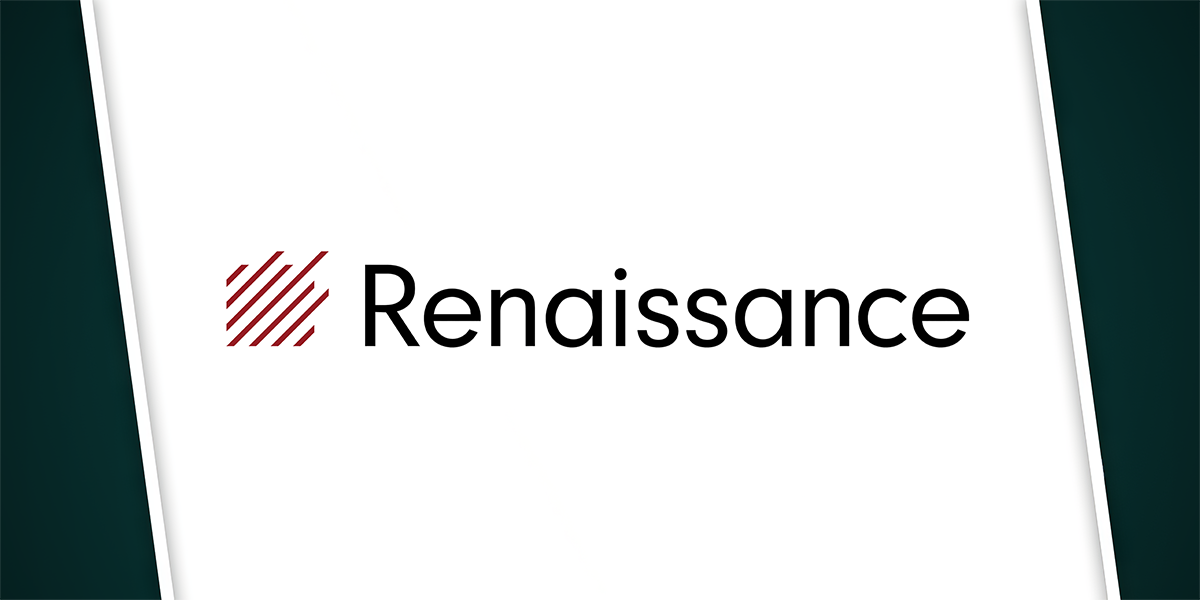
Hidden Markov Model (HMM) for Trend Prediction
I. Conceptual Overview
1. What is a Hidden Markov Model?
A Markov model assumes that a system moves between discrete “states” in a chain-like process: the probability distribution of the next state depends (only) on the current one. A Hidden Markov Model goes one step further: the states themselves are unobserved (“hidden”), and all we see are “observations” that are generated (stochastically) by whichever hidden state we’re in.
Mathematically, we say:
- \( X_t \) is the hidden state at time \( t \). It can take a finite set of values—for example, \({ S_1, S_2, \ldots, S_K }\).
- \( Y_t \) is the observation at time \( t \). Each state \( X_t \) has a probability distribution for the emission \( Y_t \).
We track two key things:
- A transition matrix \( A = [a_{ij}] \), where \( a_{ij} = P(X_{t+1}=S_j \mid X_t=S_i) \).
- An emission distribution for each state—often a Gaussian or mixture of Gaussians in real-valued data. For example, if our hidden state is “Bullish,” maybe daily returns are distributed \( \mathcal{N}(\mu_{\text{bull}}, \sigma_{\text{bull}}^2) \).
We fit an HMM using historical data for \({Y_t}\) (in our case, daily returns, or differences in log-prices). The algorithm tries to:
- Estimate the most likely parameters \((A, {\text{emissions}}, \pi_0)\).
- Infer the hidden states \(X_1, \ldots, X_T\) or at least the probability that \( X_t = S_k \) for each \( t \).
2. Applying This to “Trend Prediction”
In finance, our daily observation might be:
\[ Y_t = \text{(return on day }t\text{)}\quad \text{or} \quad Y_t = \ln\left(\frac{P_{t}}{P_{t-1}}\right) \]
Because the true “market regime” (bullish vs. bearish vs. sideways) is not directly visible, we let it be hidden. The HMM tries to cluster historical returns into states that the model deems to share similar statistical patterns.
- “Bullish” State might have a positive mean daily return,
- “Bearish” State might have a negative mean daily return,
- “Neutral” State might have low or near-zero mean returns, or higher volatility, etc.
The classic approach: once the model is fit, you observe new incoming data—like the latest day’s return—and use the HMM forward-backward or Viterbi algorithm to infer the probability the market is in each state. If the model strongly suggests “Bullish,” you take a long position (buy). If strongly “Bearish,” you might short-sell or hold inverse exposure. This is the basic trading rule.
Key Points to Understand
- Number of States: Must be chosen. Typically 2–5 for simple “regimes.”
- Emission Distribution: Commonly a GaussianHMM (each hidden state has a normal distribution of returns).
- Transition Matrix: The model learns how likely it is to remain in a bullish state vs. switch from bullish to bearish, etc.
- Signal Extraction: After fitting, we label the states by their means—e.g., the state with the largest positive mean return = “Bullish,” etc. Then for each new day’s return, we get the “most likely current state” or the “posterior distribution” over states. That becomes our signal.
Risks / Pitfalls
- Historical patterns may not persist.
- HMM-based signals can flip quickly if new data is surprising.
- Real trades must account for transaction costs, slippage, shorting constraints, etc.
II. Detailed Python Implementation
Below is a toy example using hmmlearn. Please note:
- This uses randomly simulated returns (or you can swap in real data).
- It fits a 3-state Gaussian HMM.
- It classifies each state as bullish, bearish, or neutral by mean emission.
- Then it gives a simplified “signal” for the most recent day.
Installation:
pip install numpy pandas hmmlearn
import numpy as np
import pandas as pd
from hmmlearn import hmm
# -----------------------------
# 1) Simulate or load data
# -----------------------------
# Let's pretend we have daily returns for ~1000 trading days.
# In real life, you'd load these from a CSV or an API (e.g., yfinance).
# For demonstration, let's simulate 3 "regimes":
# Regime A: Bullish ~ Normal(+0.1%, 0.5%)
# Regime B: Neutral ~ Normal(0.0%, 0.3%)
# Regime C: Bearish ~ Normal(-0.08%, 0.7%)
np.random.seed(123)
N = 1000 # number of days
hidden_states_true = []
sim_returns = []
# We'll create a random sequence of states with some "stickiness"
# so we don't jump around too rapidly.
current_state = np.random.choice([0,1,2])
for t in range(N):
# Force some regime persistence: 80% chance of staying in the same state
if np.random.rand() < 0.2:
current_state = np.random.choice([0,1,2])
hidden_states_true.append(current_state)
if current_state == 0: # bullish
r = np.random.normal(0.001, 0.005)
elif current_state == 1: # neutral
r = np.random.normal(0.0, 0.003)
else: # bearish
r = np.random.normal(-0.0008, 0.007)
sim_returns.append(r)
returns = np.array(sim_returns)
dates = pd.date_range(start="2020-01-01", periods=N, freq='B') # B=business days
df = pd.DataFrame({"date": dates, "returns": returns})
# This is the only column we feed into the HMM: each row is an "observation."
observations = returns.reshape(-1, 1)
# -----------------------------
# 2) Fit a 3-state GaussianHMM
# -----------------------------
model = hmm.GaussianHMM(n_components=3, covariance_type="full", n_iter=100, random_state=42)
model.fit(observations)
# The model will learn:
# - A 3x3 transition matrix
# - Means (mu_1, mu_2, mu_3) for the emission probabilities
# - Covariances for each state's emission distribution
# -----------------------------
# 3) Identify which state is "bullish/bearish/neutral"
# -----------------------------
# We look at each state's mean returns. The state with the largest mean
# is "bullish"; the smallest is "bearish"; and the middle is "neutral."
estimated_means = model.means_.flatten() # shape (3,)
# Sort states by their mean returns
state_order_by_mean = np.argsort(estimated_means)
bearish_state = state_order_by_mean[0] # lowest mean
neutral_state = state_order_by_mean[1] # middle mean
bullish_state = state_order_by_mean[2] # highest mean
print("Estimated means (each dimension ~ daily return):", estimated_means)
print(f" State {bullish_state} has highest mean -> BULLISH")
print(f" State {neutral_state} has middle mean -> NEUTRAL")
print(f" State {bearish_state} has lowest mean -> BEARISH\n")
# -----------------------------
# 4) Infer hidden states for entire time series
# -----------------------------
# We can do this with model.predict(...) or model.predict_proba(...)
predicted_states = model.predict(observations)
df["predicted_state"] = predicted_states
# Assign label
def label_state(s):
if s == bullish_state:
return "Bullish"
elif s == bearish_state:
return "Bearish"
else:
return "Neutral"
df["state_label"] = df["predicted_state"].apply(label_state)
# -----------------------------
# 5) Simple Trading Signal Example
# -----------------------------
# A naive approach: If today's predicted state is bullish, go LONG tomorrow;
# if bearish, SHORT tomorrow; if neutral, do nothing.
# Let's see the final day’s state and produce a "signal" for the next day.
latest_state = df["predicted_state"].iloc[-1]
latest_label = df["state_label"].iloc[-1]
if latest_state == bullish_state:
signal = "GO LONG"
elif latest_state == bearish_state:
signal = "GO SHORT"
else:
signal = "STAY OUT (NEUTRAL)"
print(f"Latest Date: {df['date'].iloc[-1].date()} \n"
f"Predicted State: {latest_label} \n"
f"Trading Signal for Next Day: {signal}")
# -----------------------------
# 6) (Optional) Evaluate or Backtest
# -----------------------------
# In real usage, you'd measure how well these signals would have performed historically,
# factoring in transaction costs. For example:
#
# - If 'Bullish' on day t, hold actual returns[t+1].
# - If 'Bearish', hold -returns[t+1].
# - If 'Neutral', 0.
#
# Then compute total PnL or other metrics.
#
# But that is an entire backtest pipeline beyond the scope of this snippet.
Notes and Directions for Further Practice
- Number of States: We chose 3 for demonstration. You can experiment with 2 or 4 (or more).
- Feature Engineering: Instead of a single daily return value, you might include e.g. volatility, volume, etc. That means each \( Y_t \) is a multi-dimensional vector. A higher-dimensional HMM can be used (still from hmmlearn with
n_features=dim
). - Real Data: Replace the simulation with actual historical equity prices. Convert them to returns, feed into the model, and see if coherent market regimes appear.
- Trade Execution: A real system would incorporate execution logic to break large orders into smaller chunks, manage risk, etc.
- Model Tuning: You can adjust
covariance_type
(full
,diag
,spherical
, etc.), number of states, or even use a GMM-HMM (Gaussian Mixture emissions).
III. Summary
Hidden Markov Models map naturally to “regime-switching” financial markets. Each hidden state corresponds to a different regime (bullish, bearish, sideways), each with its own statistical profile of returns. By estimating transition probabilities between these regimes and the likelihood of current regime membership, traders can generate daily signals (long, short, or neutral).
Although simple to conceptualize, in practice:
- Fitting requires large, clean historical datasets.
- Performance in live markets depends on whether the past patterns persist.
- Position sizing, transaction costs, and risk management are essential.
Nonetheless, HMMs remain a powerful educational tool and stepping stone to more advanced regime detection and machine learning methods in quantitative trading.
High-Frequency Statistical Arbitrage in Equities
I. Conceptual Overview
1. What is Statistical Arbitrage?
Statistical Arbitrage (“stat arb”) in equities typically involves finding small mispricings between securities (stocks, ETFs, index futures, etc.) that on average revert over short timescales. You run a large number of tiny bets simultaneously, each with a small positive expected value, with the goal of capturing consistent low-volatility returns. Because these edges are often fleeting, you typically trade frequently (intraday or even sub-second in true HFT).
A Simple Illustration: Pairs Trading
A canonical example is pairs trading. Suppose we notice two strongly correlated stocks, \(S_A\) and \(S_B\). Historically, \(S_A\) and \(S_B\) move almost in lockstep. Then, on a given day, \(S_A\) jumps up relative to \(S_B\). The assumption (backed by data) might be:
- The relationship between \(S_A\) and \(S_B\) is stable enough that large deviations are likely to mean-revert.
- We can profit by simultaneously shorting the stock that’s relatively overvalued (the “rich” one) and going long the one that’s relatively undervalued (“cheap” one).
If that spread does converge, the short position appreciates and/or the long position also appreciates. The net profit is the difference between the two. Over many such mini-opportunities across many pairs, you can produce consistent returns.
2. High-Frequency Angle
Where does “high frequency” come in?
- These relationships can change rapidly intraday as order flows push stocks around. A mispricing might last only seconds or milliseconds.
- A genuine high-frequency stat arb operation uses ultra-fast data feeds, co-location near exchanges, and optimized order execution to exploit small “spread dislocations” before others can.
However, the core mathematics behind the trades (which revolve around correlation, regression, z-score signals, etc.) is the same whether you hold for seconds or days. The main difference is speed: fast detection and execution to capture fleeting edges.
3. Mathematical / Statistical Foundations
In a typical approach:
- Stationary Spread: For a pair \((S_A, S_B)\), we might hypothesize:
\[\text{Spread}(t) \;=\; \text{Price}_A(t) \;-\; \beta \,\times\, \text{Price}_B(t)\]
where \(\beta\) is some constant regression coefficient. If this spread is stationary and mean-reverting, we can model it e.g. as an Ornstein-Uhlenbeck process or a simpler approach with z-scores. - Z-score:
\[Z(t) \;=\; \frac{\text{Spread}(t) \;-\; \mu_{\text{spread}}}{\sigma_{\text{spread}}}\]
If \(Z(t)\) is large (positive), the spread is higher than usual → short the spread (short \(S_A\), long \(S_B\)). If \(Z(t)\) is very negative, do the opposite. - Execution: In true HFT, you slice large orders into small chunks, place them on different exchanges, and do so faster than your competitors to avoid adverse selection.
In practice, a stat arb shop might run thousands of these “pairs” or “baskets” simultaneously, each with a tiny expected edge. The scale and frequency enable overall profits even if each trade has, say, a 0.05% expected return.
II. Stat Arb in Python (Toy Implementation)
Below is a simplified pairs trading script. This is not truly high-frequency—you’d need special infrastructure for that. But the code captures the core statistical logic behind a short-term “stat arb” approach.
Note: In a real high-frequency environment, you’d:
- Stream live tick data (rather than daily closes).
- Deploy minimal-latency code in C++ or similarly fast environment.
- Manage order routing and the mechanics of partial fills, cancellations, etc.
Here, we’ll:
- Load or simulate two correlated time series.
- Fit a linear relationship between them.
- Compute the “spread,” look at a z-score, and generate buy/sell signals.
- Evaluate if the strategy is profitable in an intraday sense. (We’ll do a day-level snippet for demonstration.)
import numpy as np
import pandas as pd
import statsmodels.api as sm
# -----------------------------------------------------------
# 1) Sample or load data
# For demonstration, we simulate correlated daily prices.
# -----------------------------------------------------------
np.random.seed(42)
N = 300
# We'll define a base "drift" for stock A, and a correlated stock B.
price_A = 100 + np.cumsum(np.random.normal(0, 1, N)) # random walk
# Construct stock B with correlation:
price_B = 200 + np.cumsum(0.8 * np.random.normal(0, 1, N) + 0.2*(price_A - 100)/10)
dates = pd.date_range(start="2023-01-01", periods=N, freq='B')
df = pd.DataFrame({
"date": dates,
"A": price_A,
"B": price_B
})
# In real usage, you might load from e.g. an intraday feed or yfinance:
# dfA = yfinance.download("STOCK_A", interval="1m")
# dfB = yfinance.download("STOCK_B", interval="1m")
# Then you'd merge them. For now, we proceed with the simulation.
# -----------------------------------------------------------
# 2) Fit a linear model for B ~ alpha + beta * A
# This "beta" helps define the spread = B - beta * A
# -----------------------------------------------------------
X = sm.add_constant(df["A"]) # [1, A]
model = sm.OLS(df["B"], X).fit()
alpha, beta = model.params
df["spread"] = df["B"] - (beta*df["A"] + alpha)
# Compute rolling mean and std (toy approach: entire sample or rolling window)
spread_mean = df["spread"].mean()
spread_std = df["spread"].std()
df["zscore"] = (df["spread"] - spread_mean) / spread_std
# -----------------------------------------------------------
# 3) Generate signals based on z-score
# If z-score > +1, short B & long A
# If z-score < -1, long B & short A
# -----------------------------------------------------------
entry_z = 1.0
exit_z = 0.0 # exit if zscore returns to near 0
df["position"] = 0 # +1 means "spread is too low => short A, long B"
# -1 means "spread is too high => long A, short B"
# We'll use a simple state machine approach:
current_pos = 0
for i in range(N):
z = df.loc[i, "zscore"]
if current_pos == 0:
# No position currently
if z > entry_z:
# spread is "too high" => B is expensive relative to A
current_pos = -1
elif z < -entry_z:
# spread is "too low" => B is cheap relative to A
current_pos = 1
else:
# Already have a position
# If zscore crosses exit threshold near 0, close
if (current_pos == -1 and z < exit_z) or (current_pos == 1 and z > -exit_z):
current_pos = 0
df.at[i, "position"] = current_pos
# "position" here is from the perspective of the spread.
# If position=+1 => we are effectively long B, short A.
# -----------------------------------------------------------
# 4) PnL Calculation (naive daily approach)
# We'll shift the positions by 1 day to reflect we hold next day's changes
# -----------------------------------------------------------
df["pos_shifted"] = df["position"].shift(1).fillna(0)
# Daily returns:
# if we are +1 on spread => daily PnL ~ + (dB - beta*dA)
# if we are -1 on spread => daily PnL ~ - (dB - beta*dA)
df["retA"] = df["A"].pct_change()
df["retB"] = df["B"].pct_change()
# Approx spread returns each day:
df["spread_ret"] = df["retB"] - beta * df["retA"]
df["strategy_ret"] = df["pos_shifted"] * df["spread_ret"]
df["cum_return"] = (1 + df["strategy_ret"]).cumprod()
print(df[["date","A","B","spread","zscore","position","strategy_ret","cum_return"]].tail())
# Evaluate final cumulative return:
final_cum_ret = df["cum_return"].iloc[-1]
print(f"\nFinal Cumulative Return from Spread Strategy: {final_cum_ret - 1:.2%}")
Interpreting the Results
- Beta ~ The slope from the regression that tries to linearly relate
B
toA
. - Spread = \( B - (\beta \times A + \alpha) \). If it’s high, that implies
B
is “too expensive” vs.A
. - Z-score normalizes that spread to standard deviations from the mean.
- Positions reflect the sign of the bet on that spread. A short spread means short B and long A. A long spread means the opposite.
- PnL arises if the spread reverts back toward zero.
Note: In a high-frequency context, you’d apply exactly the same formula but to intraday data (1-minute, 1-second, or even real-time ticks). You’d also attempt to place limit orders or join the order book, possibly capturing the bid-ask spread.
III. Key Considerations in Real High-Frequency Trading
-
Execution Speed & Infrastructure:
- Co-locate servers in data centers near exchanges to reduce round-trip latency.
- Use specialized market data feeds (e.g., direct feeds vs. slower consolidated feeds).
- Implement auto-cancel and partial-fill logic to avoid adverse selection.
-
Order Types:
- Market orders risk paying wide spreads.
- Limit orders can add complexity about fill probability and queue position.
-
Risk & Leverage:
- Even though each trade has a small edge, you typically leverage to amplify returns.
- Must watch out for “black swan” events or regime changes that break your correlation assumptions.
-
Regulatory & Fee Structure:
- Exchange fees, maker-taker rebates, short-locate costs—these matter heavily in HFT.
-
Profit from “Microstructure”:
- Some HFT stat arb is about quickly detecting price dislocations across multiple venues (e.g., two exchanges quoting different prices for the same stock).
- The theoretical “arbitrage” is near risk-free if done instantly.
IV. Summary
High-Frequency Statistical Arbitrage in equities relies on:
- Identifying short-lived mispricings (often spread relationships).
- Quickly entering offsetting trades that bet on mean reversion or convergence.
- Repeating thousands of times daily with minimal latency.
The math behind it is straightforward linear regression, correlation, z-scores, and stationarity tests—concepts familiar to someone with an undergraduate math background. The complexity and barrier to entry primarily come from:
- Technical infrastructure (ultra-fast computing + exchange connectivity).
- Operational discipline (risk management, cost control).
The above code snippet is a toy demonstration of the core ideas. Real HFT shops simply push them to extreme scale and extreme speed.
Multi-Factor “One Model” for Global Markets
I. Conceptual Overview of a Multi-Factor “One Model”
1. Multi-Factor Alpha Extraction
a) Multi-Factor Basics
A traditional “factor model” approach in equities might say:
\[\text{Return}_i(t+1) = \beta_1 \times \text{Momentum}_i(t) \;+\; \beta_2 \times \text{Value}_i(t) \;+\; \dots\]
where each \(\beta_j\) is the weight assigned to a “factor” (e.g., momentum, value, volatility, etc.). Typically, you would fit these factors for one region (say, U.S. equities) and keep it separate from other asset classes.
b) “One Model” Extends This Across All Assets
Instead of a separate factor model for U.S. stocks, a second for European stocks, a third for FX, etc., we feed everything into one machine learning system that sees, for example:
- Equities: Past price returns, volume, fundamentals (P/E, cash flow, etc.), technical indicators, plus cross-asset features (like bond yields, commodity prices).
- FX: Exchange rate changes, carry, interest rate differentials, plus broad macro signals (like equity vol, net capital flows).
- Commodities: Seasonality, inventory data, shipping rates, implied volatility.
- Bonds: Yield curve signals, inflation data, central bank announcements.
- …and so on.
The “One Model” approach tries to find global patterns. Perhaps an equity price can partly be predicted by certain moves in oil, or interest rates, or certain currency crosses. By training on all of these simultaneously, the model can discover subtle correlations.
2. Feature Engineering and Data Structure
For each instrument \(i\) on each day \(t\), you collect a vector of features:
\[X_{i,t} = \bigl(x_{i,t}^{(1)}, x_{i,t}^{(2)}, \dots, x_{i,t}^{(d)}\bigr)\]
where each \(x_{i,t}^{(j)}\) might be a function of:
- The security’s own time series (past returns, moving averages, RSI, etc.).
- Related macro data (oil price, yield curve shape).
- Data from other securities or indices (S&P 500, VIX, etc.).
You also gather a “target” variable, e.g. next-day (or next-hour) return of instrument \(i\):
\[y_{i,t} = \frac{P_{i,t+1} - P_{i,t}}{P_{i,t}}\]
The model tries to learn:
\[y_{i,t} \approx f\bigl(X_{i,t}\bigr)\]
for all instruments \(i\), all times \(t\). This yields a single fitted function \(f(\cdot)\).
3. Trading Decisions
After fitting \( f \), each day you can:
- Predict each instrument’s next-day return \(\hat{y}_{i,t+1}\).
- Sort or rank instruments by \(\hat{y}_{i,t+1}\).
- Allocate capital to the top predicted winners (long) and possibly short the worst ones.
- Or feed those predicted returns into an optimizer that also includes constraints on risk, liquidity, etc.
The key difference from multiple independent models is that all instruments/markets see each other’s data, and you have a single integrated set of predictions. This can let the model exploit cross-asset relationships better.
4. Potential Advantages & Pitfalls
- Advantage: If there is a strong correlation between, say, a jump in USDJPY currency pair and technology stocks, a unified model can pick that up better than two siloed models.
- Pitfall: Complexity. A single model with huge dimensional data (thousands of instruments × dozens/hundreds of features each) can be tough to manage, clean, and interpret. Overfitting is a concern.
In practice, Renaissance Technologies and other advanced quants invest heavily in clean data and infrastructure for this approach. They run one massive codebase that ingests all signals, updating them in near real-time, and outputs a single set of buy/sell instructions.
II. Toy Python Implementation
Below is a simplified demonstration of how one might structure a “One Model” pipeline for multi-asset daily returns. We will:
- Construct a DataFrame of features for multiple tickers in multiple asset classes.
- Fit a RandomForestRegressor that tries to predict next-day returns.
- Generate signals from the model’s predictions.
Disclaimer: This toy code is for conceptual demonstration. Actual “One Model” systems use advanced ML algorithms, specialized infrastructure, live data feeds, robust risk management, etc.
import numpy as np
import pandas as pd
from sklearn.ensemble import RandomForestRegressor
from sklearn.model_selection import train_test_split
# ------------------------------------------------------
# 1) Create or load multi-ticker, multi-asset data
# We'll simulate a small dataset here.
# ------------------------------------------------------
np.random.seed(123)
N = 500 # 500 trading days
symbols = ["AAPL", "XOM", "USDJPY", "Gold", "SP500"] # eq, eq, FX, commodity, index
all_rows = []
for sym in symbols:
# Simulate a random walk price
base = 100 if sym not in ["USDJPY", "Gold", "SP500"] else 50
noise_scale = 1.0
prices = base + np.cumsum(np.random.normal(0, noise_scale, N))
# We'll create some random 'factors'
factor1 = np.random.normal(0, 1, N) # e.g. momentum
factor2 = np.random.normal(0, 1, N) # e.g. volume-based signal
factor3 = np.random.normal(0, 1, N) # e.g. some cross-asset or fundamental
# Next-day return target
# For demonstration, let's just take the daily returns shifted -1
returns = np.diff(prices, prepend=prices[0]) / prices[:-1]
# Shift by 1 so we have "tomorrow's return" as target
future_returns = np.roll(returns, -1)
future_returns[-1] = 0 # last day has no future return
for t in range(N):
all_rows.append({
"date": t, # or actual dates
"symbol": sym,
"price": prices[t],
"factor1": factor1[t],
"factor2": factor2[t],
"factor3": factor3[t],
"next_ret": future_returns[t]
})
df_all = pd.DataFrame(all_rows)
# Example final shape: each row is (day, symbol, factors, next-day-return)
print(df_all.head(10))
# ------------------------------------------------------
# 2) Prepare data for a "One Model"
# We'll just treat each (symbol, day) as a separate example
# ------------------------------------------------------
# In real usage, you might add cross-asset features: e.g. SP500 price for
# *all* rows. For demonstration, keep it simple.
features = ["factor1", "factor2", "factor3"]
target = "next_ret"
X = df_all[features].values
y = df_all[target].values
# We'll do a simple train/test split (not time-series correct, but for demonstration)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, shuffle=True)
model = RandomForestRegressor(n_estimators=100, random_state=42)
model.fit(X_train, y_train)
r2_train = model.score(X_train, y_train)
r2_test = model.score(X_test, y_test)
print(f"Train R^2: {r2_train:.3f}, Test R^2: {r2_test:.3f}")
# ------------------------------------------------------
# 3) Generate signals from the model
# We'll simply rank each (symbol, day) by predicted next_ret
# ------------------------------------------------------
df_all["predicted_ret"] = model.predict(df_all[features])
# For demonstration, let's pick a single day
test_day = 300
df_today = df_all[df_all["date"] == test_day].copy()
df_today = df_today.sort_values("predicted_ret", ascending=False)
print("\nPredictions for day =", test_day)
print(df_today[["symbol", "predicted_ret"]])
# Suppose we go long top 2 predicted returns, short bottom 2
# This is a naive, example "One Model" daily tactic.
long_symbols = df_today["symbol"].iloc[:2].tolist()
short_symbols = df_today["symbol"].iloc[-2:].tolist()
print(f"Go LONG on: {long_symbols}, SHORT on: {short_symbols}")
Explanation of the Code
- Data Generation
We create a random-walk style “price,” some random factor signals (factor1
,factor2
, etc.), and define “next_day_return” as the training target. - Single Model
- We flatten everything into a big dataset: each row = (symbol, day, factor1, factor2, factor3, next_ret).
- A real system might add cross-asset features. E.g., the row for AAPL might also include the current price of USDJPY or the current difference in interest rates.
- Prediction
- We train a
RandomForestRegressor
to predict next-day return from the factor signals. - The same model is used across all symbols. That is the “One Model” concept.
- We train a
- Signal
- On a given day, we predict next-day returns for all symbols.
- We pick the top predicted returns to go long and the bottom predicted returns to short.
In a real pipeline, you’d run:
- Daily (or intraday if you want short horizons):
- Update all factor values (momentums, volatilities, fundamentals, cross-asset data).
- Infer predictions from the single model.
- Rebalance the portfolio or place orders.
III. Additional Considerations
-
Cross-Asset Features:
In a real “global one-model” approach, each row might have dozens (or hundreds) of features spanning multiple asset classes. E.g. “today’s VIX,” “today’s gold price change,” “interest rate differential,” etc. -
Dimensionality & Overfitting:
- The more features you add, the more data you need.
- In practice, firms invest heavily in data cleaning, building GPU clusters, and orchestrating data flows.
-
Portfolio Optimization:
- Once the model outputs a predicted return (alpha) for each instrument, a separate process (like a quadratic optimizer) might handle how to size positions, subject to constraints (risk targets, liquidity, leverage, etc.).
-
Execution:
- Real-time signals → trade in an automated fashion.
- Large volumes → watch out for market impact.
IV. Summary
A Multi-Factor “One Model” aggregates signals across all asset classes, geographies, and instruments into a single integrated prediction engine. This holistic approach can capture subtle correlations in global markets. Though the math behind each feature is typically straightforward (momentum, spreads, macro data, etc.), the engineering and infrastructure are extremely complex:
- You need broad data coverage—equities, FX, commodities, macro.
- You must unify all these data streams into one codebase, one pipeline, one model.
- You have to carefully handle risk, slippage, and execution once the model decides which assets to long/short.
Firms like Renaissance Technologies have demonstrated how powerful a well-designed “One Model” can be. It requires major scale, robust QA for data, and continuous R&D—but can yield extraordinary returns if the signal detection remains strong.
Leveraging & Execution Tactics (“Basket Options” Conceptual Demo)
I. Conceptual Overview
1. Leverage and Derivatives
A core idea in quantitative trading is to amplify small statistical edges by trading larger positions than your raw capital would allow. This can be done through:
- Margin: Borrowing cash or securities to trade more shares.
- Futures or Swaps: Gaining synthetic exposure to an underlying (equity index, interest rate, commodity) at a fraction of its notional cost.
- Options: Non-linear payoffs (calls, puts) that can produce high effective leverage.
a) Why Leverage?
If you believe your strategy has a stable, positive expected return, you want to apply leverage to boost the absolute dollar gains. The main risk is that drawdowns become magnified, so risk management is crucial.
2. “Basket Options”
A basket option is a derivative whose payoff depends on a weighted combination (basket) of multiple underlying assets. For instance, let
\[B_t = w_1 S_1(t) \;+\; w_2 S_2(t) \;+\; \dots \;+\; w_n S_n(t),\]
where \(S_i(t)\) is the price of asset \(i\) at time \(t\) and \(w_i\) are the weights. A basket call option might give its holder the right to buy this entire basket at a certain strike \(K\), with payoff at maturity \(T\) of:
\[\max\bigl(B_T - K, \, 0\bigr).\]
This single derivative effectively packages multiple assets, providing:
- Convenience: One contract to get combined exposure.
- Tax or Regulatory Nuances: Sometimes the specific structure offers benefits or “work-arounds” in certain jurisdictions.
- Leverage: An option is inherently leveraged (you pay the option premium, not the full cost of the basket).
Historical Note
Renaissance Technologies (as discussed in the “basket option” context) allegedly used such structures partly to transform short-term trading gains into longer-term capital gains. Regardless of legal ramifications, the financial essence was: use a derivative that references a basket of underlying positions, letting the fund effectively control a larger notional size with fewer capital or regulatory constraints.
3. Execution Tactics
Whether or not you’re trading via “basket options,” the broad challenge is:
- How to buy or sell large amounts of underlying assets (or derivative contracts) without moving the price or tipping off competitors.
a) Order Slicing
A large buy (or sell) order can be divided into many small child orders (“slices”) and executed over time. This aims to:
- Reduce market impact: fewer big block trades that shift price.
- Disguise overall size from high-frequency front-runners.
b) Algorithmic Execution
Professional trading platforms implement advanced execution algorithms (VWAP, TWAP, POV, etc.), all of which revolve around systematically distributing trades to minimize cost or reveal as little as possible.
II. Python Implementation: Basket Payoff + Execution Slicer
Below is a toy example illustrating:
- A “basket call option” payoff via simple Monte Carlo simulation.
- Slicing a large order for partial fills.
Disclaimer: This code is purely educational. Actual usage in real markets involves more advanced math, live feeds, risk controls, etc.
import numpy as np
# ---------------------------------------------------------------------
# 1) Simulate correlated price paths for multiple assets in a "basket"
# ---------------------------------------------------------------------
np.random.seed(42)
n_assets = 3 # number of assets in the basket
n_sims = 10_000 # number of Monte Carlo simulations
T = 1.0 # time horizon in years
r = 0.01 # risk-free rate (for discounting, if we wanted)
sigma = [0.2, 0.25, 0.3] # volatilities for each asset
corr = 0.5 # assume moderate correlation between assets
# Current prices
S0 = np.array([100.0, 50.0, 150.0])
# Covariance matrix (simple approach: same corr among all pairs)
cov_matrix = np.zeros((n_assets, n_assets))
for i in range(n_assets):
for j in range(n_assets):
if i == j:
cov_matrix[i, j] = sigma[i]**2
else:
cov_matrix[i, j] = corr * sigma[i] * sigma[j]
# Weights for the basket
weights = np.array([0.5, 1.0, 0.8]) # e.g. weighting the assets differently
strike = 200.0 # strike for the entire basket call
# Generate correlated random draws for each simulation
L = np.linalg.cholesky(cov_matrix) # Cholesky decomposition for correlation
dt = T # one-step to maturity
rand_norm = np.random.normal(size=(n_sims, n_assets))
corr_shocks = rand_norm.dot(L.T)
# Geometric Brownian motion style: S_T = S0 * exp( (r - 0.5*sigma^2)*T + sigma*sqrt(T)*Z )
# We'll do a single-step approximation for demonstration
final_payoffs = []
for sim in range(n_sims):
# For each asset, compute final price
ST = []
for i in range(n_assets):
drift = (r - 0.5 * sigma[i]**2) * dt
diffusion = sigma[i] * np.sqrt(dt) * corr_shocks[sim, i]
price_T = S0[i] * np.exp(drift + diffusion)
ST.append(price_T)
ST = np.array(ST)
# Basket value at maturity
basket_val = (ST * weights).sum()
# Basket call payoff
payoff = max(basket_val - strike, 0.0)
final_payoffs.append(payoff)
# Expected payoff (ignoring discount factor for simplicity)
option_price_est = np.mean(final_payoffs)
print(f"Estimated Basket Call Price ~ {option_price_est:.2f}")
# ---------------------------------------------------------------------
# 2) Execution Slicer: Breaking a large order into smaller chunks
# ---------------------------------------------------------------------
def slice_order(total_size, n_slices):
"""
Simple function to slice a large order into smaller pieces.
For a real system, you'd factor in real-time price, liquidity, etc.
"""
size_per_slice = total_size // n_slices
orders = []
for i in range(n_slices):
orders.append({
"slice_number": i,
"trade_size": size_per_slice
})
# If there's a remainder leftover, put it in the last slice
remainder = total_size % n_slices
if remainder != 0:
orders[-1]["trade_size"] += remainder
return orders
# Suppose we want to buy 15,000 units of the basket (or derivative)
# We'll break it into 10 slices
large_order = 15000
sliced_orders = slice_order(large_order, 10)
print("\nSliced Orders:")
for o in sliced_orders:
print(o)
Explanation
-
Basket Option Pricing (Monte Carlo)
- We assume each asset follows a simple Geometric Brownian Motion with correlation.
- After one step to maturity \(T\), we sum up \( w_i \times S_i(T) \) to get the basket value.
- We compute the payoff = \(\max(\,\text{basket} - \text{strike},\, 0)\) for a call.
- The average payoff across many simulations is the undiscounted approximate fair value.
-
Order Slicing
- We illustrate a trivial function
slice_order(...)
to break a large order (15,000 units) into 10 smaller trades. In a real environment:- You’d incorporate timing (e.g., place each slice over a period of minutes)
- You’d check partial fills, queue position, limit vs. market orders, etc.
- We illustrate a trivial function
III. Final Takeaways
- Leverage allows a trader to magnify returns (and risks) when statistical edges are believed to be stable.
- Basket Options (or other structured derivatives) can grant leveraged exposure to multiple assets at once—often with special legal/tax properties.
- Execution Tactics matter enormously for large trades. Order slicing and algorithmic execution help reduce market impact and detection by competitors.
Ultimately, the ability to structure derivatives (like basket options) plus advanced execution is a big part of how sophisticated quant firms scale their strategies. They capture small edges, amplify them with leverage or derivative overlays, and implement trades in a stealthy, cost-efficient manner.